Reverse List Order
Table of Contents
Reversing a list is a common task in programming and data processing. It helps users organize information from bottom to top. This technique works well in text manipulation, data cleanup, and automation tools.
As a CSE engineer, I often build tools that help non-tech users with everyday tasks. One of these is a tool that lets users reverse the order of list items. I will explain how to build it and why it matters.
What Is a List?
A list is a group of items written one after another. Each item usually appears on a new line. Lists help users manage tasks, store data, or present steps.
Example of a List
Apple
Banana
Cherry
Date
This list contains fruits in their original order. Reversing the list will show:
Date
Cherry
Banana
Apple
Why Reverse a List?
People reverse lists for many reasons. Writers use it to change the structure of articles. Programmers use it for sorting data. Users may want to see the most recent item first. This task saves time and adds value in daily workflows.
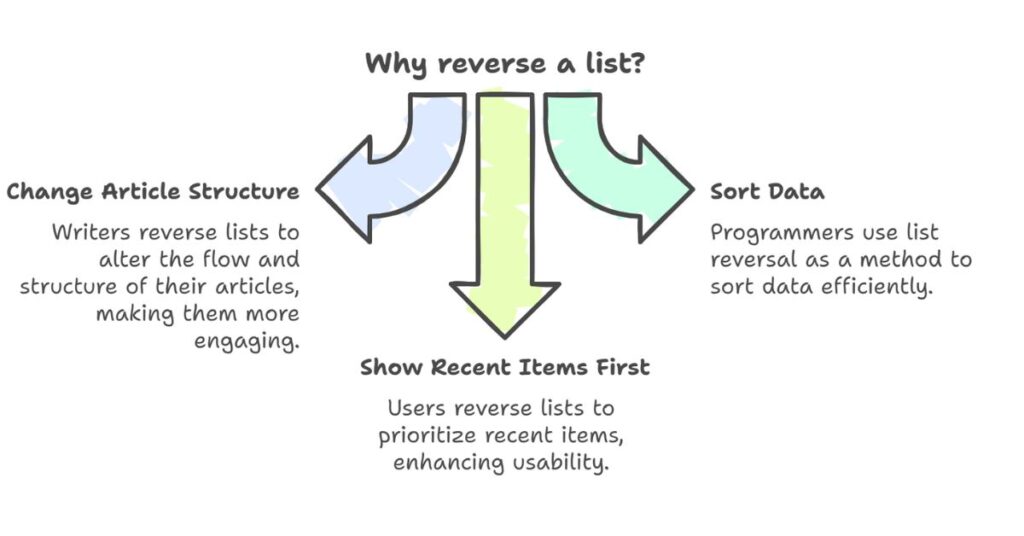
Where Can You Use It?
Reversing a list works well in many areas:
- To-do apps: Show the last task first
- Programming: Change data order in arrays
- Data entry: Fix input mistakes
- Text editing: Reformat bulleted or numbered lists
Common Use Cases in IT
1. Code Debugging
Programmers reverse logs to find recent errors quickly.
2. Data Handling
Data analysts reverse CSV or TXT rows to update entries.
3. Web Development
Front-end developers reverse item lists in user interfaces.
How to Reverse a List Manually
Manual list reversal is easy. Follow these steps:
- Copy your list.
- Paste it into a text editor.
- Select all lines.
- Use reverse line tools or scripts.
- Copy the output and use it.
This method is simple but slow for large files.
Use JavaScript to Reverse a List
As a CSE engineer, I prefer automation. JavaScript can reverse a list fast and reliably.
Example Code
function reverseList(text) {
const lines = text.trim().split('\n');
return lines.reverse().join('\n');
}
You pass your list as a string. The code returns the reversed list.
Create a Web Tool
You can build a simple web tool using HTML, CSS, and JavaScript. Add a text box for input and output. Include buttons for reverse, copy, paste, and clear.
Key Features
- Input area
- Reverse button
- Copy button
- Paste button
- Refresh button
- Output area
This tool helps users without coding skills.
SEO and UI Tips for Web Tools
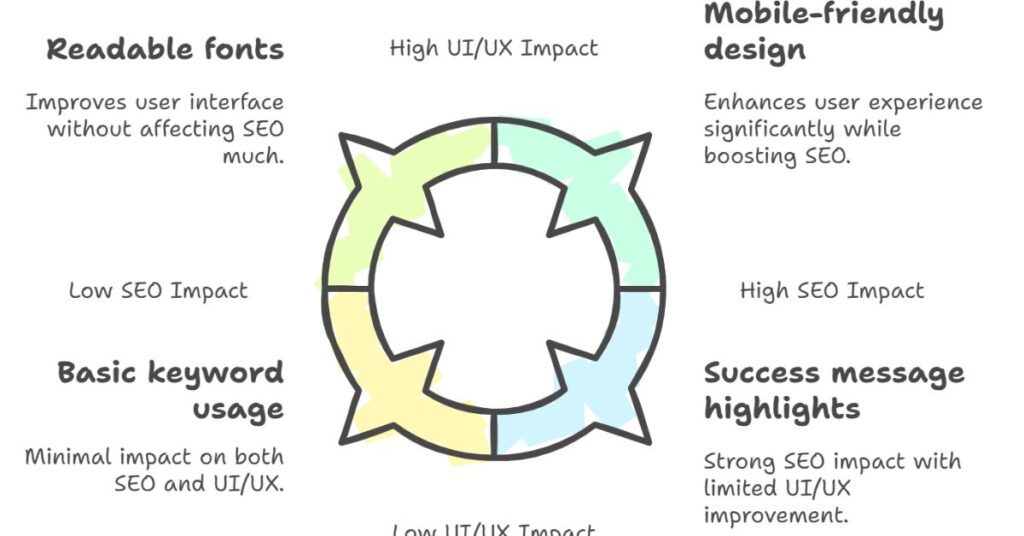
1. Use Keywords
Add keywords like “reverse list”, “flip list order”, and “reverse lines” to improve ranking.
2. Optimize UI
Use readable fonts and clean layouts. Make the tool mobile-friendly.
3. Improve UX
Add alerts and placeholders. Highlight success messages after copy or paste.
Challenges You May Face
1. Empty Lines
Some users include empty lines. Filter them out before reversing.
2. Extra Spaces
Trim lines to avoid spacing issues.
3. Clipboard Access
Browsers may block paste without user permission. Handle errors with alerts.
Final Thoughts
Reversing a list is a basic but useful feature. It works in many fields. Programmers, writers, and office workers use it often. I build these tools as a developer to help others work faster and wiser.
This task shows how small changes can save time. You can write a script or build a tool for repeated use. If you want to try it, use the code above or the online tool.