Extract Emails from Text
Table of Contents
What Does It Mean to Extract Emails?
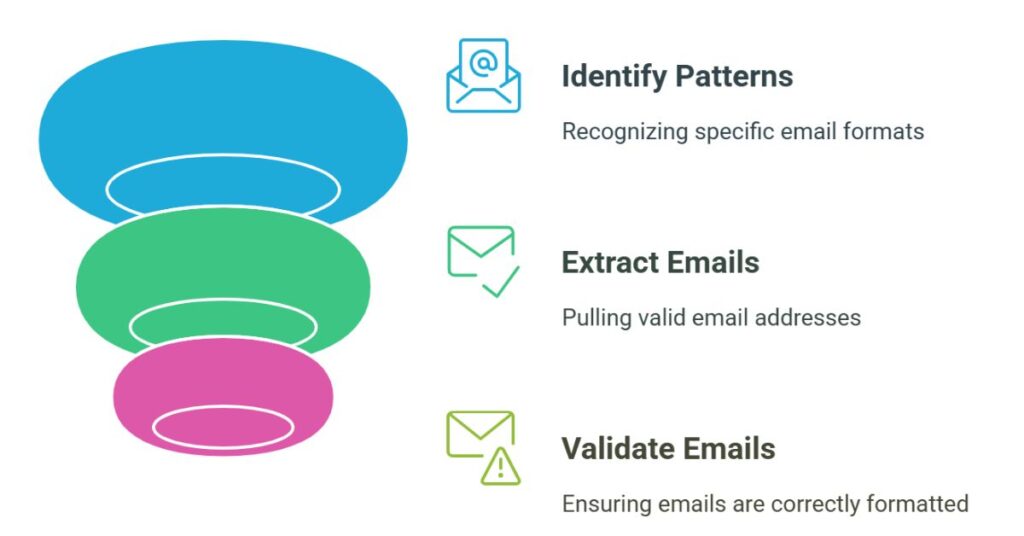
Email extraction means pulling valid email addresses from a block of text. The text may come from a document, website, chat, or database. We look for specific patterns that match email formats like user@example.com
.
Why Is Email Extraction Important?
Many teams use email extraction for marketing, customer support, and data collection. If you work in IT, you may need to gather emails from logs or text files. Manual extraction is slow and prone to errors. Automation helps save time and ensures accurate results.
Common Use Cases
Marketing
Marketers collect emails from leads and inquiries. They use this data to send newsletters or updates.
Customer Support
Support teams extract emails from tickets or chats. They reply quickly and solve user problems.
Research and Data Analysis
Data analysts extract emails to study user behavior or contact participants.
How Email Extraction Works
Email extraction uses pattern matching. A common method uses regular expressions (regex). Regex helps find email-like patterns from text.
Example of Regex Pattern
lessCopyEdit[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-z]{2,}
This pattern finds most valid emails. It matches common formats like john.doe@example.com
.
Tools to Extract Emails from Text
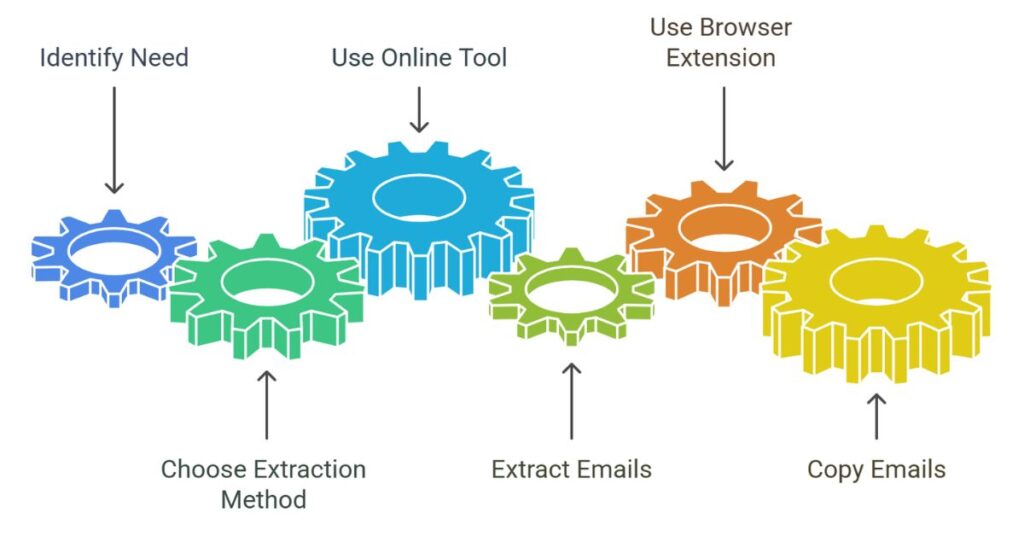
There are many ways to extract emails. You can use software, online tools, or write code.
Use Online Tools
Online tools let users paste text and extract emails. These tools are fast and easy. Some free tools are:
- Email Extractor by Small SEO Tools
- Extract Emails by ToolsBug
- Online Email Extractor by TextMechanic
Paste your text, click a button, and get your list.
Use Browser Extensions
Some Chrome extensions can extract emails from web pages. These include:
- Email Extractor by DevRain
- Hunter.io Chrome Extension
You visit a site, click the extension, and copy the emails.
How to Extract Emails with Code
As a developer, you may want to extract emails using code. Here is a basic example using JavaScript:
const text = "Contact us at info@example.com or support@company.net";
const emails = text.match(/[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-z]{2,}/g);
console.log(emails);
Explanation
text.match(...)
finds all matching email patterns.- The regex checks for valid emails.
- The result is an array of email addresses.
This method works in the browser or Node.js environment.
How to Extract Emails Using Python
Python is another good option for this task. Here is a simple example using Python:
import re
text = "Send queries to help@domain.com or admin@site.org"
emails = re.findall(r"[a-zA-Z0-9._%+-]+@[a-zA-Z0-9.-]+\.[a-z]{2,}", text)
print(emails)
How It Works
re.findall()
finds all matches in the text.- The pattern is the same as in JavaScript.
- You get a list of emails in return.
Tips for Better Email Extraction
Remove Unwanted Text
Clean the text before running the tool. Remove line breaks, extra spaces, or symbols.
Validate Emails
Some tools extract wrong patterns. Use validation to check if emails are real.
Avoid Duplicates
Filter the result to remove duplicate emails.
Export Data
Save the results in .txt
or .csv
format for future use.
How to Use HTML Tools for Extraction
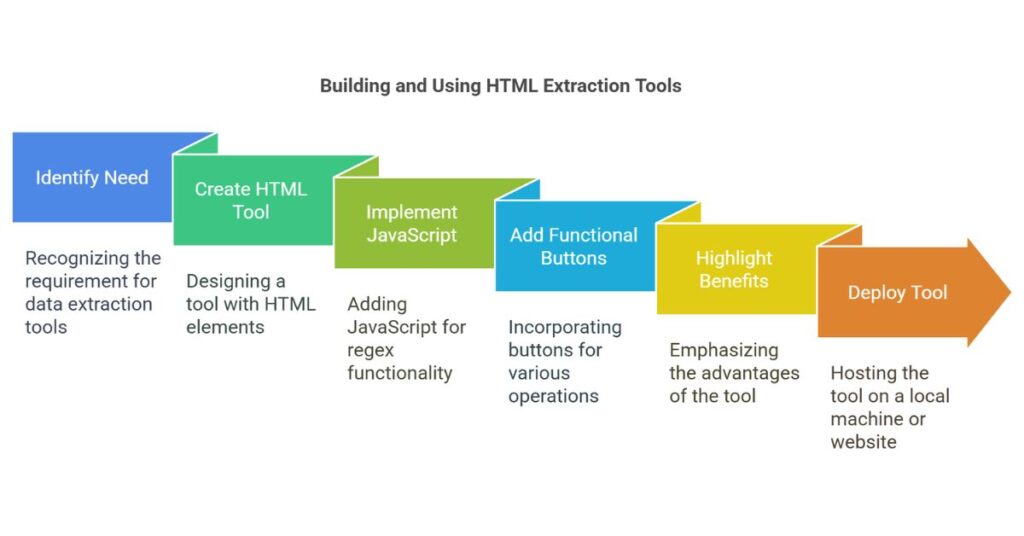
You can build a simple HTML tool for this task. Use <textarea>
for input and output. Use JavaScript to run the regex. Add buttons to convert, copy, paste, save, and reset.
Benefits of HTML Tools
- Works offline
- Runs in the browser
- Easy to use for non-technical users
You can host it on a local machine or website.
Use Cases in Real Projects
In one of my projects, I had to extract over 1,000 emails from website comments. I built a tool using JavaScript and HTML. It saved hours of manual work. The tool handled bulk text and returned clean email lists.
Another time, I worked with a database of support tickets. I wrote a Python script to collect emails from the message body. The client used the list for follow-up communication.
SEO and Technical Benefits
Using this method improves productivity. It reduces human error. For developers, it means a better workflow. For businesses, it means faster data handling. These benefits help in IT, customer service, and sales operations.
This process also improves SEO when used on web forms. If your site captures valid emails, your mailing list grows. More emails mean more reach.
Conclusion
Email extraction is a simple yet useful task. It helps in marketing, support, and development. Tools and scripts make it easy to extract emails from large texts. As a CSE engineer, I suggest using regex and automation for best results. Use clean code and simple logic to handle this task effectively. Always validate and store results safely.