How to Convert a List to a Semicolon-Separated Format
Table of Contents
What is a Semicolon-Separated Format?
A semicolon-separated format is a way to list items in one line using semicolons. This format helps reduce line breaks and makes data easier to read for machines. Many developers use it when working with databases, CSV files, or configuration files.
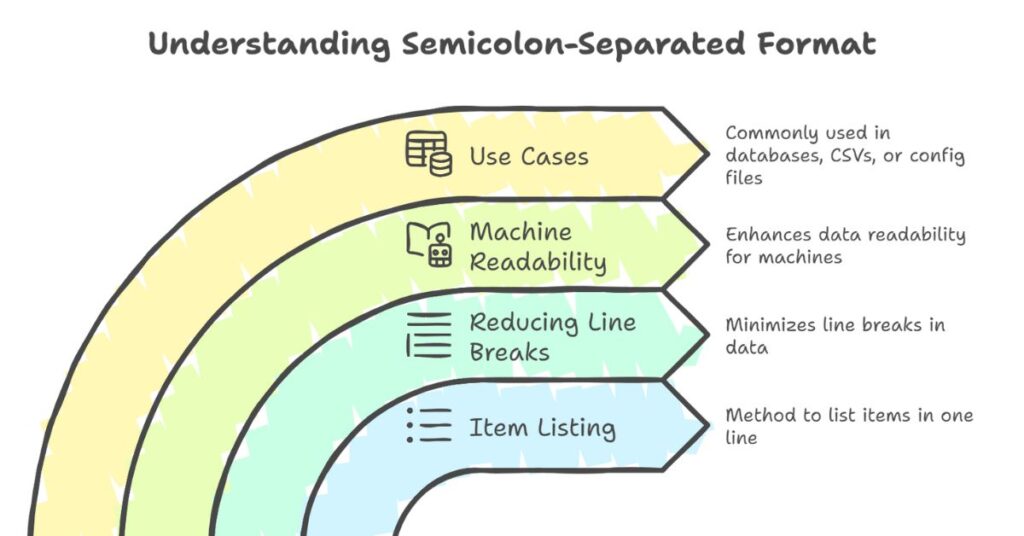
Example:
Apple; Banana; Mango; Orange
Why Do We Use a Semicolon Instead of a Comma?
Sometimes, the list items already contain commas. In that case, using commas to separate items can confuse the system. A semicolon helps avoid that confusion. It is also useful in systems that accept semicolon-separated values instead of commas.
Use Case in the IT Sector
In the IT sector, we often use semicolon-separated lists in:
- Environment variable files
- Data export tools
- Database scripts
- CSV import with special delimiters
- Input forms that accept bulk entries
For example, if a developer submits a list of tags in a web form, converting them into a single semicolon-separated line helps the backend process the data faster.
Step-by-Step: Convert a List to Semicolon Format
Step 1: Prepare the List
Write your list with one item per line. It can be in a text file or inside a text box on a web tool.
Example:
Dog
Cat
Rabbit
Fish
Step 2: Remove Empty Lines
Empty lines can cause errors. Make sure there are no blank lines between the items. Trim any space before or after each item.
Step 3: Join Items with Semicolon
Now, you need to combine all items using a semicolon. The result should look like this:
Dog; Cat; Rabbit; Fish
Step 4: Use an Online Tool
You can use our simple HTML tool at /blog/convert-list-to-semicolon/. It supports:
- Paste list
- Convert to semicolon format
- Copy output
- Download as a file
This tool helps non-technical users convert lists quickly without writing code.
Convert a List Using JavaScript (For Developers)
If you are a developer, you can convert a list using a few lines of JavaScript.
const list = `Dog
Cat
Rabbit
Fish`;
const result = list.split(/\r?\n/).map(item => item.trim()).filter(item => item).join("; ");
console.log(result); // Output: Dog; Cat; Rabbit; Fish
This method works well for front-end applications or Node.js scripts.
Convert a List Using Python
Python is widely used in the IT sector. You can use the join() method to convert lists.
lines = ["Dog", "Cat", "Rabbit", "Fish"]
result = "; ".join(lines)
print(result) # Output: Dog; Cat; Rabbit; Fish
You can also read from a file and write the output to another file.
Benefits of Semicolon Format
- It saves space by reducing line breaks.
- It works better with systems that support custom delimiters.
- It improves data readability when exporting to Excel or CSV.
- It supports multilingual or special character entries more cleanly than commas.
Common Errors and Fixes
Extra Spaces
Problem:
Apple ; Banana ; Mango
Fix:
Trim spaces before and after each item before joining.
Empty Items
Problem:
An empty line creates a double semicolon.
Fix:
Filter out empty lines using filter(item => item !== “”).
Double Semicolon
Problem:
Apple;;Banana
Fix:
Avoid joining lists with empty values. Clean data before joining.
When to Avoid Semicolon Format
Avoid using semicolons when:
- The system only accepts comma-separated values
- You use JSON or XML format
- The list is large and needs a structured format like an array or table
Tools That Help You Convert Easily
Several tools can help you convert lists:
- Our HTML converter
- Notepad++ with regex
- Excel’s TEXTJOIN formula
- VSCode with Find and Replace
These tools speed up your work and reduce manual errors.
Final Thoughts
As a CSE engineer, I often deal with data formatting issues. Converting lists to a semicolon-separated format is simple but useful. Whether you’re a developer or a non-technical user, this method helps you keep your data clean and machine-readable. Use tools or code, but always clean your input before converting. This habit will improve your workflow and reduce future bugs.
Leave a Reply