Extract Numbers from Text
Table of Contents
What Does Extracting Numbers Mean?
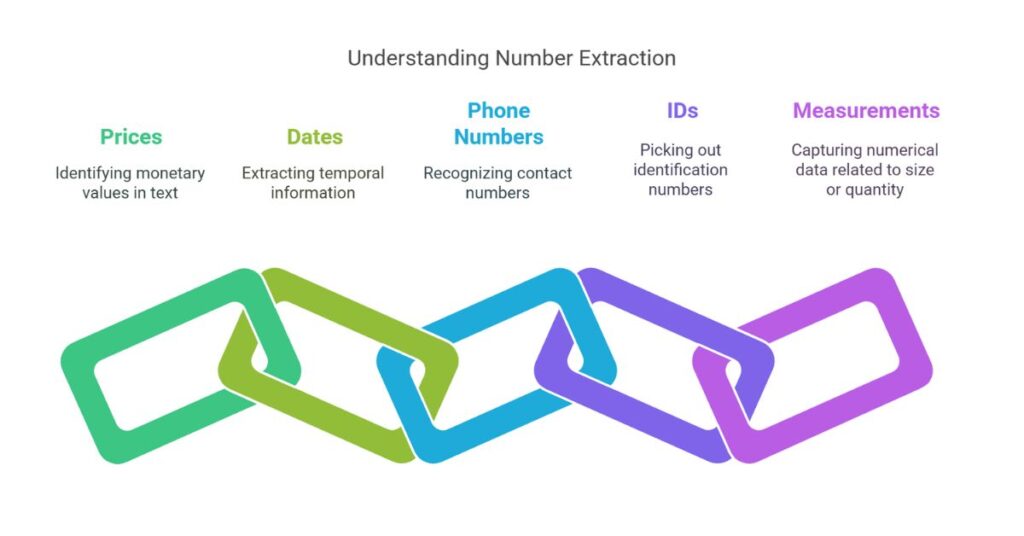
Extracting numbers means picking out digits or number patterns from a block of text. This text can come from emails, documents, logs, forms, or websites. Numbers may include prices, dates, phone numbers, IDs, or measurement values.
Why Do We Need to Extract Numbers?
We extract numbers to process them in software systems. Numbers help track user input, calculate results, analyze data, or create reports. For example:
- A billing system extracts amounts from invoices.
- A survey system pulls age or rating values.
- A web scraper collects prices from websites.
All of these need a clean and accurate way to fetch numeric values from text.
Common Sources of Text With Numbers
Numbers appear in many types of text. Here are a few common examples:
- Emails: “Your order #12345 has been shipped.”
- Logs: “Memory used: 256MB.”
- Web pages: “Price: $49.99 per item.”
- Forms: “Enter your 10-digit mobile number.”
To work with such data, we must use code or tools that extract the numbers.
Tools to Extract Numbers From Text
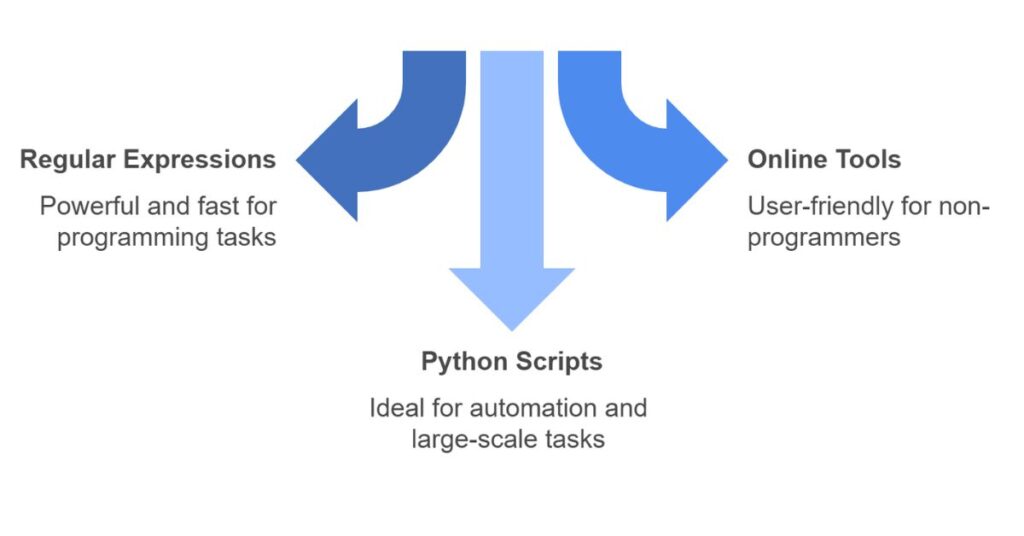
You can use many tools and methods to extract numbers from text. I use them based on the use case.
1. Regular Expressions (Regex)
Regex is a powerful way to find patterns in text. In programming, you can use a pattern like /\d+/g
to match all integers. You can also use /\d+(\.\d+)?/g
to match both whole and decimal numbers.
Example in JavaScript:
let text = "Order ID 452 and price is $25.75";
let numbers = text.match(/\d+(\.\d+)?/g);
console.log(numbers); // Output: ["452", "25.75"]
This is fast and works well in most languages like Python, JavaScript, and PHP.
2. Online Number Extractor Tools
Many online tools let you paste your text and extract numbers. These tools are useful for non-programmers.
Example Tool Features:
- Paste your input.
- Click a button to convert.
- Copy or save the result.
I built one using HTML, JavaScript, and CSS with features like Copy, Paste, Save File, and Reset. These tools help in quick manual processing.
3. Using Python Scripts
Python is good for text tasks. It has built-in support for regex. Here’s a simple example:
import re
text = "Invoice #9898, Total: $300.50"
numbers = re.findall(r'\d+\.?\d*', text)
print(numbers)
Python is helpful in automation and large-scale data extraction jobs.
How to Handle Different Number Formats
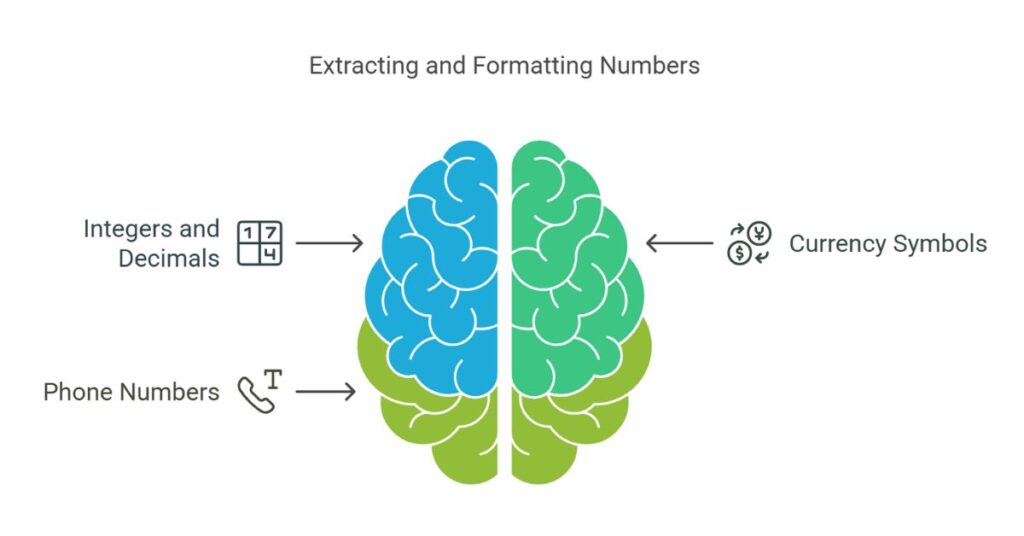
1. Integers and Decimals
Extract both whole and decimal numbers using proper regex.
- Integer:
\d+
- Decimal:
\d+\.\d+
2. Currency Symbols
If numbers come with $
, €
, or ৳
, you can remove the symbol after extraction or adjust your regex to skip it.
Example: $49.99
→ 49.99
3. Phone Numbers
Phone numbers often include +
, -
, or spaces. Use a pattern like /\+?\d[\d\s-]+/g
to extract them correctly.
Use Cases in the IT Sector
As a software engineer, I apply number extraction in many systems.
1. Web Scraping Projects
We extract product prices, review counts, and ratings from e-commerce sites.
2. Form Validation
We validate phone numbers or zip codes from user input.
3. Log Analysis
We extract CPU, RAM usage, or error codes from system logs.
4. Data Cleaning
In data science, we clean mixed text and pull numeric values for further analysis.
Step-by-Step Summary
Step 1: Get the input text
Paste or load the text from a document, form, or site.
Step 2: Use a method or tool
Use regex, an online converter, or a script.
Step 3: Match number patterns
Focus on digits, decimals, or phone formats.
Step 4: Output the result
Display, copy, or save the extracted numbers.
Final Thoughts
Extracting numbers from text is a basic but important skill in IT. As a CSE engineer, I use it in real-world software tasks. It helps in automation, data cleaning, and backend logic. You can use simple tools or code to get it done. Once you learn the pattern, the process becomes very easy and fast.
Whether you’re building a web app, writing a script, or analyzing data, extracting numbers is a handy skill that saves time and effort.