Extract Hashtags from Text
Table of Contents
Hashtags are widely used in social media to organize and categorize content. They help users find relevant topics or discussions based on a specific keyword or trend. Extracting hashtags from text can be helpful in various applications, such as social media management, data analysis, and marketing.
Why Extract Hashtags from Text?
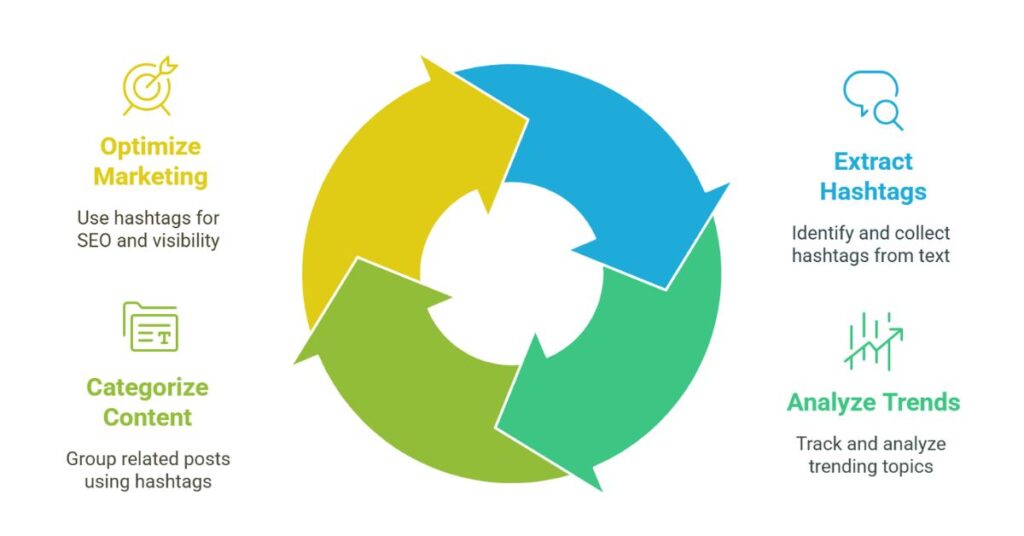
Hashtags are keywords or phrases prefixed with the symbol #
. They are commonly used on platforms like Twitter, Instagram, and LinkedIn to make content discoverable. Extracting hashtags from text can be beneficial for several reasons:
- Social Media Analysis: By extracting hashtags, you can track trending topics and analyze the reach of specific keywords.
- Content Categorization: Hashtags help categorize content. Extracting them allows you to group related posts.
- Marketing and SEO: Hashtags improve visibility on social media. Extracting them can help with SEO optimization and marketing strategies.
Understand the Basic Structure of a Hashtag
A hashtag begins with the symbol #
, followed by a word or phrase without spaces. For example, #DataScience
or #AIInTech
are valid hashtags. It’s important to note that hashtags are typically case-insensitive, which means #AI
and #ai
refer to the same topic. When extracting hashtags, we focus on identifying these patterns within the text.
Tools for Extracting Hashtags
There are different methods and tools you can use to extract hashtags from text. Some common tools include:
- Regular Expressions: Regular expressions (regex) are a powerful tool for pattern matching. You can use regex to find hashtags in any text input.
- Programming Languages: Languages like Python and JavaScript offer libraries to help extract hashtags from text.
For instance, in Python, the re
module can be used to find hashtags by matching the #
symbol followed by letters and numbers.
Extract Hashtags Using Regular Expressions
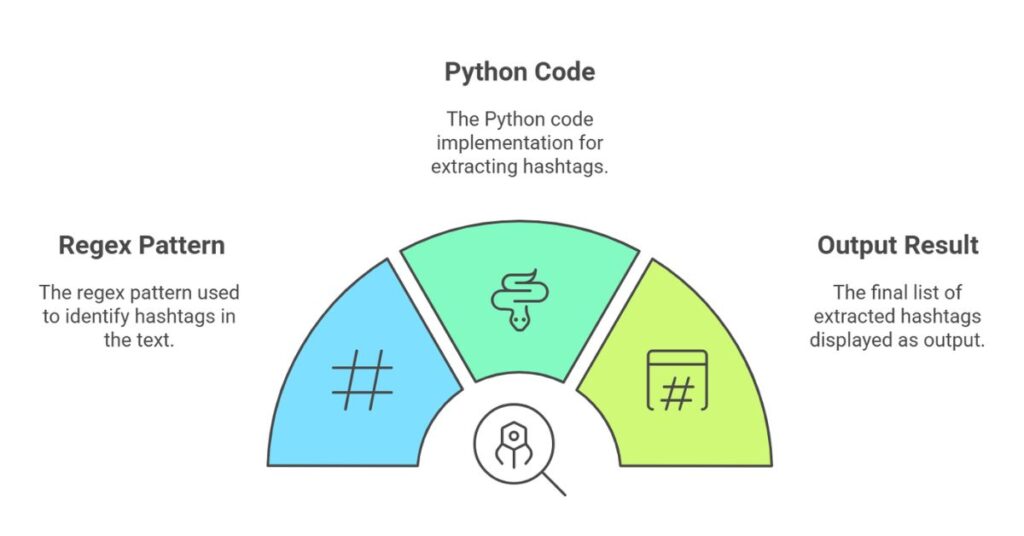
Regular expressions provide a simple and efficient way to extract hashtags from text. Below is a basic example of how to use regex in Python to extract hashtags:
import re
# Sample text
text = "Learning Python is fun! #Python #Programming #AI"
# Regex pattern to match hashtags
hashtags = re.findall(r'#\w+', text)
# Display the hashtags
print(hashtags)
Explanation:
r'#\w+'
: This pattern matches a#
symbol followed by one or more word characters (letters, numbers, and underscores).re.findall()
: This function returns all occurrences of the pattern in the text.
In this example, the output will be ['#Python', '#Programming', '#AI']
.
Extract Hashtags in JavaScript
JavaScript can also be used for extracting hashtags from text, especially when working on web applications. Here’s a simple JavaScript function to extract hashtags:
function extractHashtags(text) {
var regex = /#\w+/g;
var hashtags = text.match(regex);
return hashtags;
}
var text = "Learning JavaScript is fun! #JavaScript #WebDevelopment #Tech";
console.log(extractHashtags(text));
Explanation:
/\#\w+/g
: This regex pattern matches hashtags similar to the Python example.match()
: This JavaScript method returns an array of matches found in the string.
The output of this function will be ["#JavaScript", "#WebDevelopment", "#Tech"]
.
Practical Applications of Hashtag Extraction
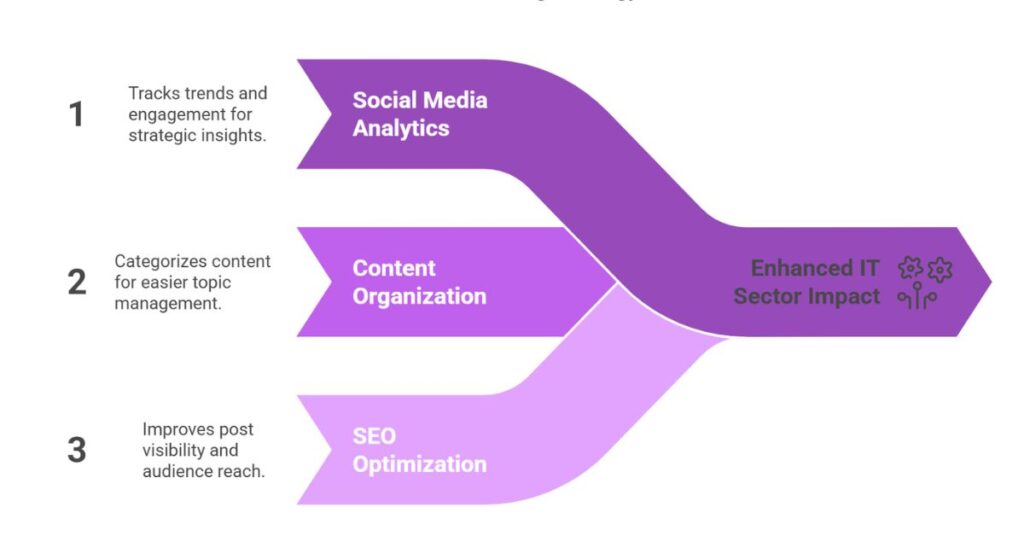
Hashtags have many applications in the IT sector. Here are some practical uses of extracting hashtags:
1. Social Media Analytics
By extracting hashtags from social media posts, you can track popular topics, identify trends, and monitor user engagement. This data can be helpful for content creators, marketers, and businesses.
2. Content Organization
Hashtags are a simple way to categorize content. For instance, by extracting hashtags from a collection of articles, you can group them into topics such as #Technology
, #Marketing
, or #AI
.
3. SEO Optimization
Hashtags are important for SEO on social media platforms. Extracting and analyzing hashtags can help improve the visibility of posts. By using the right hashtags, content can reach a larger audience, enhancing its impact.
Handling Common Issues When Extracting Hashtags
While extracting hashtags from text, you might face some common issues:
- Case Sensitivity: Hashtags are case-insensitive, so you must treat
#AI
and#ai
as the same. - Special Characters: Some hashtags may contain special characters like underscores (
_
) or numbers. Make sure your extraction method handles these cases. - Multiple Hashtags: In some cases, a single post may contain multiple hashtags. Ensure your method can handle extracting multiple hashtags efficiently.
Best Practices for Hashtag Extraction
Here are some best practices when extracting hashtags:
- Use Efficient Code: Optimize your code for performance, especially when dealing with large volumes of text.
- Regular Expressions: Use regex patterns that match hashtags accurately, considering edge cases like special characters.
- Handle Multiple Hashtags: Ensure your extraction method can handle and return multiple hashtags correctly.
Conclusion
Extracting hashtags from text is an essential skill for IT professionals, marketers, and social media analysts. With simple tools like regular expressions in Python or JavaScript, you can efficiently extract hashtags and use them for various purposes, such as social media analysis, content organization, and SEO optimization. By following the steps outlined in this guide, you can start extracting hashtags from text with ease and apply them effectively in your workflow.