Tab Delimiter Converter
Table of Contents
A tab delimiter is a character used to separate values in a dataset, often in text files. It is commonly used in tab-separated values (TSV) files. This format is simple and easy to understand. It allows data to be organized in columns, making it easy to process. In this article, we will discuss the concept of tab delimiters, their uses, and how to handle them in programming.
What is a Tab Delimiter?
A tab delimiter is a special character that separates values in a text-based dataset. It is usually represented by the Tab key (ASCII code 9). The tab character is invisible but plays a crucial role in formatting data. In a TSV file, each line represents a row of data, and each column is separated by a tab character.
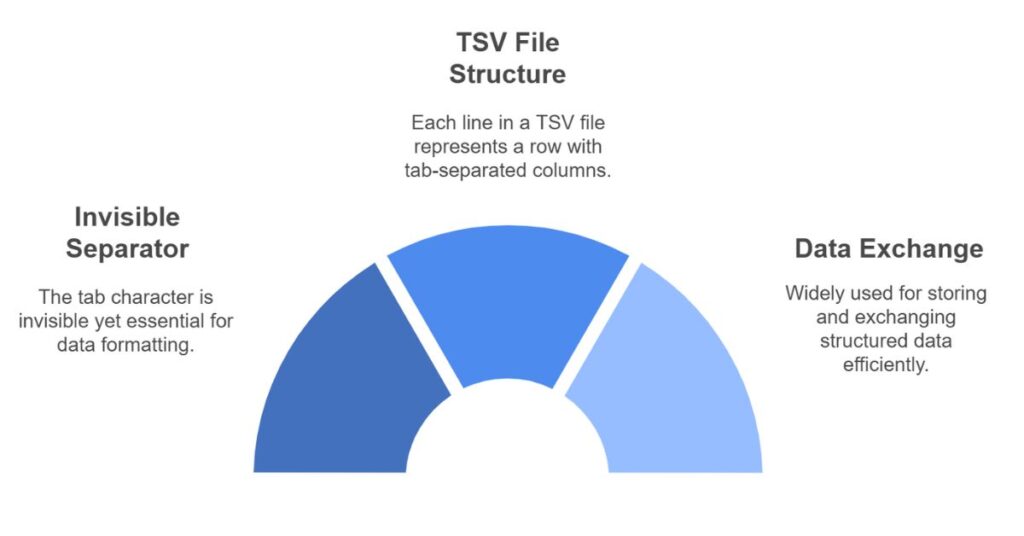
This method is widely used in programming and data processing. It is simple to use and works well for storing and exchanging structured data.
Uses of Tab Delimiters
Tab delimiters are used in various areas, especially in data processing and programming. Here are some key uses:
Data Exchange
Tab-delimited files are often used to store data that needs to be exchanged between different systems. Since the tab character is a simple and common delimiter, these files are easy to generate and read. They are widely used in industries like finance, healthcare, and research to share structured data.
Importing and Exporting Data
Tab-delimited files are commonly used for importing and exporting data in applications like Excel, Google Sheets, and databases. These applications support TSV files, allowing users to convert and import data seamlessly.
Data Analysis
In data analysis, tab delimiters are used to organize large datasets. Tools like Python and R can read and manipulate TSV files for analysis. The tab delimiter allows for quick processing and easy extraction of data from text files.
How to Use Tab Delimiters
Creating a Tab-Delimited File
Creating a tab-delimited file is simple. You can use a text editor or a spreadsheet program to create one. In a text editor, just press the Tab key between values. Each line represents a row of data, and the values in that row are separated by the tab character.
In a spreadsheet program like Microsoft Excel or Google Sheets, you can save the file as a .tsv file. The program will automatically insert tab characters between the columns.
Reading a Tab-Delimited File
To read a tab-delimited file, you need to use a program or script that supports TSV files. Python, for example, provides built-in libraries to read tab-delimited files. Here’s a simple example using Python:
# Python code to read a TSV file
with open('data.tsv', 'r') as file:
for line in file:
values = line.strip().split('\t')
print(values)
This code opens a file called data.tsv
, reads each line, and splits the values by the tab character. The split('\t')
method separates the data into columns.
Converting Tab-Delimited Data
Converting tab-delimited data into another format is easy. You can convert a TSV file into CSV or JSON format using various tools and scripts. For example, in Python, you can convert a TSV file to CSV using the following code:
import csv
# Convert TSV to CSV
with open('data.tsv', 'r') as tsv_file, open('data.csv', 'w', newline='') as csv_file:
tsv_reader = csv.reader(tsv_file, delimiter='\t')
csv_writer = csv.writer(csv_file)
for row in tsv_reader:
csv_writer.writerow(row)
This script reads a tab-delimited file and writes it into a CSV file.
Benefits of Using Tab Delimiters
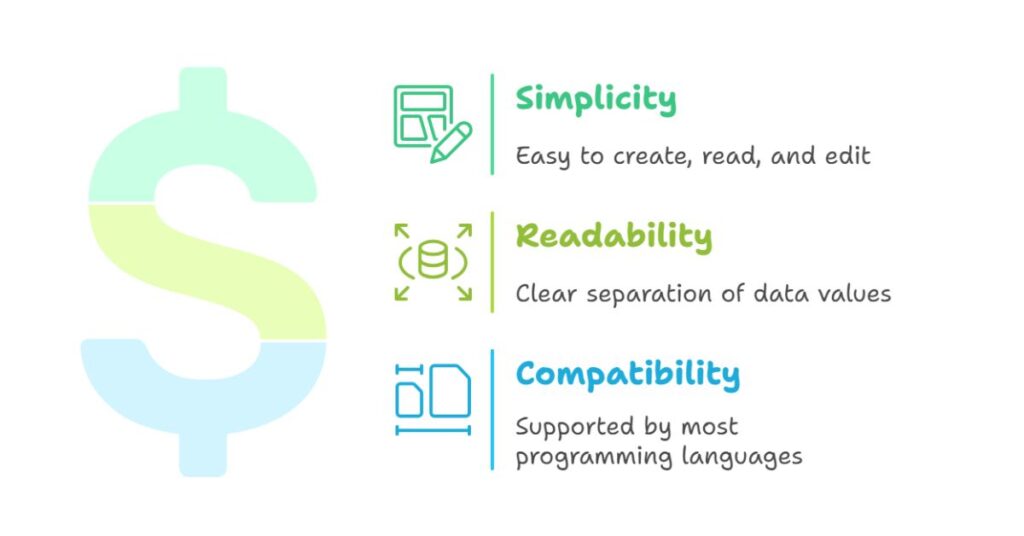
Simplicity
Tab-delimited files are easy to create, read, and edit. They are simpler than other formats like CSV or JSON, making them ideal for quick data manipulation. The tab character is not commonly used in data, so it minimizes the risk of conflicts.
Readability
Tab-delimited files are easy to read. Since each value is separated by a visible tab space, it’s simple to identify individual pieces of data. This makes it easy to troubleshoot and debug data processing issues.
Compatibility
Most programming languages and tools support tab-delimited data. For instance, Python, Java, and R all provide built-in support for handling TSV files. This makes tab-delimited data highly compatible with a wide range of applications.
Tab Delimiters vs Other Delimiters
Tab delimiters are often compared to other delimiters like commas and spaces. Each delimiter has its advantages and drawbacks.
Tab vs Comma
Comma-separated values (CSV) is another common format used for data storage. Unlike tab delimiters, commas are more likely to appear in data, leading to potential conflicts. While CSV is widely used, tab-delimited files are often preferred when data contains commas.
Tab vs Space
Space is another delimiter used in data formatting. However, spaces can sometimes be part of the data itself, leading to confusion. Tab characters, being less common in text, avoid this issue and are less likely to cause problems in data processing.
Challenges with Tab Delimiters
While tab delimiters are simple and effective, there are a few challenges:
Visibility
The tab character is invisible, making it difficult to spot errors in the data. If a tab character is missing or added incorrectly, it can cause issues when processing the data. To avoid this, always verify the integrity of the data before processing.
Handling Data with Tabs
If the data contains tab characters, it can lead to unexpected results. For instance, if the data includes a tab in a string field, it might split the string into multiple parts. To handle this, ensure that the data is sanitized and cleaned before using it.
Conclusion
Tab delimiters are a powerful tool for organizing and processing data. They are simple to use, compatible with most programming languages, and provide an efficient way to store and exchange data. Despite some challenges, tab-delimited files remain a popular choice for data processing and are widely used in industries around the world. By understanding how to use and manage tab-delimited data, you can improve your data workflows and streamline your data processing tasks.