Convert List to Space Separator
Table of Contents
Converting a list of items into a space-separated string is a simple task in programming and data processing. In many situations, you might need to turn data entered as a list (with one item per line) into a single line with each item separated by a space.
This article will guide you through how to perform this conversion using basic tools and programming techniques. Whether you are working with plain text or in a development environment, this process is valuable for text formatting, data cleaning, and many other applications in IT.
Why Convert Lists to Space Separators?
Converting a list to a space separator is important in various scenarios. Data often comes in formats that are not directly usable for further processing. For example, you may receive a list of items, one per line, but need to store or display the data as a single string. The space separator format is a common method used to join individual list items without extra characters like commas or line breaks. This method is widely used in software development, web development, and data processing tasks. By learning how to convert lists to space-separated strings, IT professionals can streamline the workflow and save time.
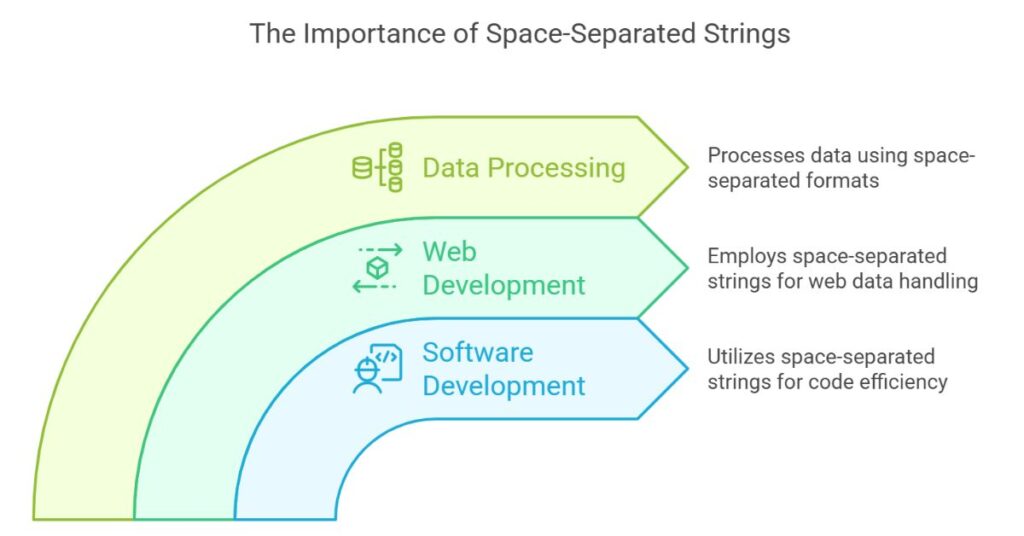
How to Convert a List to a Space Separator?
There are several methods to convert a list to a space separator. Depending on the programming language or tools you are using, the approach can vary. Below, we will walk through the most common ways to achieve this.
Using a Text Editor
If you are working with plain text and need a quick solution, a text editor can help you convert a list into a space-separated format.
- Open a text editor (such as Notepad or Sublime Text).
- Copy the list of items, making sure each item is on a new line.
- Replace line breaks with spaces: Most text editors have a find-and-replace function. In the “Find” field, type
\n
(for a newline) and in the “Replace” field, type a space. - Save the file after the replacement.
This method is quick and works well when dealing with short lists. However, for larger datasets or when working in programming environments, using code is often more efficient.
Using JavaScript
In web development, JavaScript is a powerful tool for manipulating text. You can use it to convert a list into a space-separated string. Here is a simple example:
function convertListToSpaceSeparator() {
const inputText = document.getElementById("inputText").value;
const outputText = inputText.split('\n').join(' ').trim();
document.getElementById("outputText").value = outputText;
}
In this JavaScript example:
split('\n')
breaks the input into an array by splitting at each newline character.join(' ')
joins the array into a single string, using spaces as the separator.trim()
removes any extra spaces before or after the string.
This method is very efficient for use in web applications where users need to perform the conversion dynamically.
Using Python
Python is another excellent tool for this task, especially when working with larger datasets or automating processes. The following Python code demonstrates how to convert a list of items into a space-separated string:
def convert_list_to_space_separator(input_list):
return ' '.join(input_list)
# Example usage
input_list = ['apple', 'banana', 'cherry']
output = convert_list_to_space_separator(input_list)
print(output) # Output: "apple banana cherry"
In this Python example:
join()
is used to combine the list items with a space.- This method is simple and can be used in scripts to handle lists of any size.
Python is especially useful when processing large amounts of data, as it can be automated and integrated into scripts that handle data from various sources.
Practical Applications of Space-Separated Lists
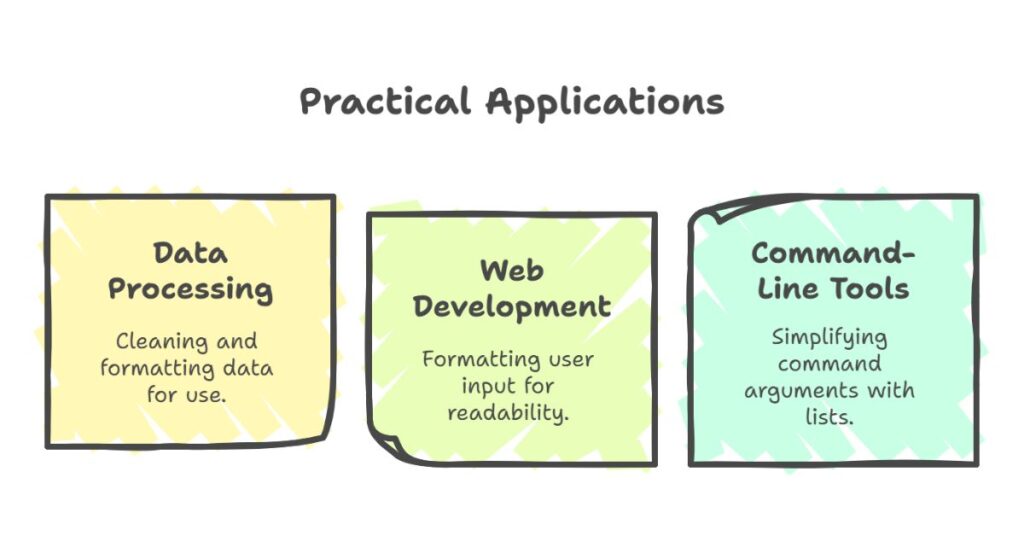
Once you know how to convert a list to a space separator, you can apply this technique in many different areas. Here are some practical examples:
Data Processing
In data processing, lists often need to be cleaned and formatted before they can be used. Converting data into a space-separated format makes it easier to work with. For example, when working with large datasets in CSV format, it might be necessary to convert lists of items into a format that can be parsed more easily.
Web Development
Web developers often need to format user input or data fetched from APIs into a readable form. Converting lists into space-separated strings can help in various situations, such as displaying tags, categories, or keywords on a website.
Command-Line Tools
When using command-line tools, you often deal with lists of files or items. Converting these lists to a space-separated format allows you to pass multiple items as arguments in a command. For example, using a space-separated list in a shell command can simplify tasks like file manipulation or batch processing.
Tools to Help with Conversion
If you’re not a programmer or don’t want to write code, there are online tools available that can help you convert a list to a space-separated string. These tools are user-friendly and allow you to paste your list, hit a button, and receive the result immediately. Some tools even allow you to copy the result to the clipboard or paste data directly from your clipboard.
Conclusion
Converting a list to a space-separated string is a simple but effective task in many IT-related fields. Whether you are working with plain text or coding in a development environment, there are many ways to achieve this conversion. Knowing how to do this can save you time and help with data processing, web development, and command-line tools. As technology evolves, understanding how to manipulate and format data efficiently will remain an essential skill for IT professionals. With this guide, you now have the tools and knowledge to handle list-to-space separator conversions quickly and effectively.