Convert CSV to List
Table of Contents
What is CSV?
CSV stands for Comma-Separated Values. CSV files store data in plain text. Each line contains values separated by commas. Many software tools use CSV to import and export data. Programmers, data analysts, and engineers often use CSV files for working with large datasets.
Why Convert CSV to List?
Many applications require data in list format. A list helps in easy manipulation and readability. When you convert CSV to a list, you make data processing easier. It also helps in debugging and displaying data.
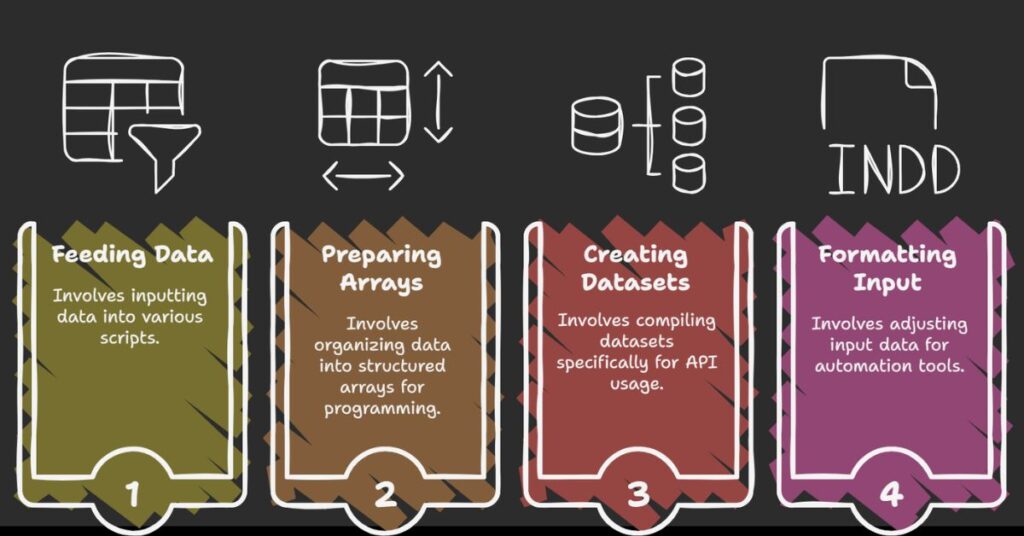
As a CSE engineer, I use CSV files to store data from forms, sensors, or APIs. But to work on this data, I first convert it to a list. This step simplifies the process and improves performance.
Common Use Cases
- Displaying user data on a webpage
- Reading student records in an app
- Importing product information into an e-commerce site
- Processing sensor data in real time
Step-by-Step Process to Convert CSV to List
Step 1: Get the CSV Data
The first step is to get the raw CSV data. You can copy it from a spreadsheet or export it from a tool. Make sure the data is clean. Check for unwanted spaces or missing values.
Example CSV:
Name, Age, City
John, 28, New York
Sara, 25, London
Alex, 30, Paris
Step 2: Split the Rows
Next, split the data by line breaks. Each line becomes one row in the list. In JavaScript, you can use split('\n')
or split(/\r?\n/)
. This converts the block of CSV text into separate rows.
Code Snippet:
const rows = csvData.trim().split(/\r?\n/);
Step 3: Split by Comma
Now split each row by a comma. This gives you individual values. Each value is an element in the list.
const list = [];
rows.forEach(row => {
const values = row.split(',');
values.forEach(val => list.push(val.trim()));
});
Step 4: Show the List
After converting, you can display the list in a text area or console. Use <br>
to separate the list items if needed.
Output Example:
Name
Age
City
John
28
New York
Sara
25
London
Alex
30
Paris
Using a Web-Based CSV to List Tool
Paste the CSV
You paste the CSV text into an input box. This step is easy and saves time. The tool takes care of the formatting.
Click Convert
Clicking the Convert button processes the text. The script splits the CSV and shows the result.
Copy or Download the List
You can copy the list or use it elsewhere. This feature helps developers save time.
Refresh and Paste Functions
The Paste button pulls content from the clipboard. The Refresh button clears the form. These make the tool user-friendly and fast.
Benefits of CSV to List Conversion
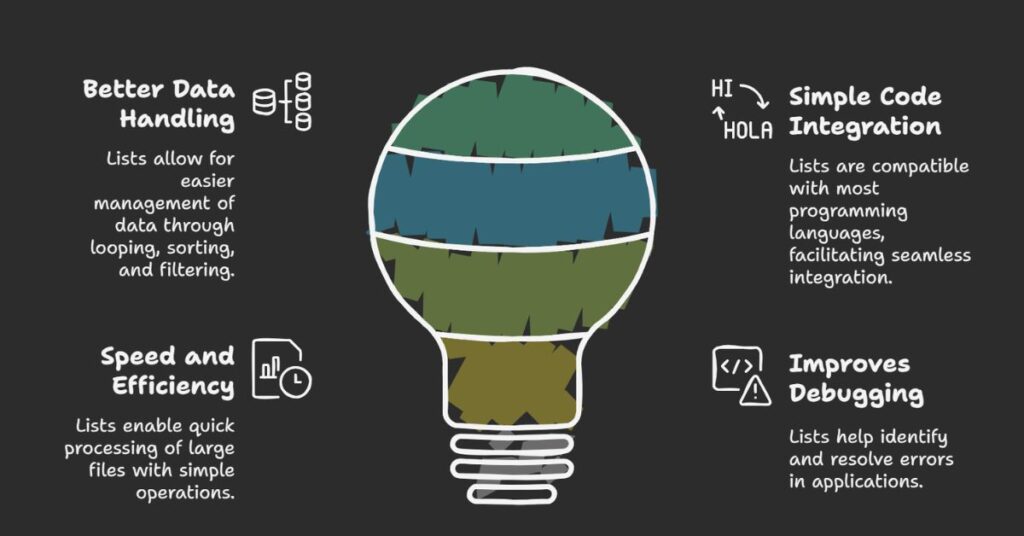
Better Data Handling
Lists are easier to manage. You can loop over them, sort them, and filter them. Working with lists improves data accuracy.
Simple Code Integration
Lists work well with most programming languages. You can use them in JavaScript, Python, PHP, or Java.
Speed and Efficiency
Using a list reduces the need for complex operations. You can process large files quickly with simple loops.
Improves Debugging
If your application shows errors, converting data to a list can help you find the problem.
Tips for Better CSV Conversion
- Remove empty lines from the CSV
- Check for missing commas
- Use
.trim()
to clean white spaces - Always test small chunks first
- Watch out for commas inside quotes
Programming Languages That Support CSV to List
avaScript
Use split()
and trim()
to turn CSV text into a list. JavaScript is good for frontend tools.
Python
Use csv.reader()
or pandas
to read CSV. Convert it to a list using loops or list comprehension.
import csv
with open('file.csv') as f:
reader = csv.reader(f)
data = []
for row in reader:
data.extend(row)
PHP
Use fgetcsv()
to read each row. Then, use array_merge()
to flatten it into a single list.
SEO Tips for CSV to List Tool
- Use keywords like CSV Converter, CSV to List Tool, and Convert CSV Online
- Add meta descriptions with your target phrases
- Write clear titles with <h2> tags
- Use tool descriptions for extra traffic
- Add FAQs about CSV formats and conversion steps
Final Thoughts
As a CSE engineer, I use CSV files every day. Turning CSV into a list helps me simplify my work. Whether I’m working with APIs, databases, or user input, this step saves time. The right tool or code can make this task easy. You can also build your tool using JavaScript. Try it, test it, and improve your data workflow today.